Patterns with Async call : Beautiful Javascript
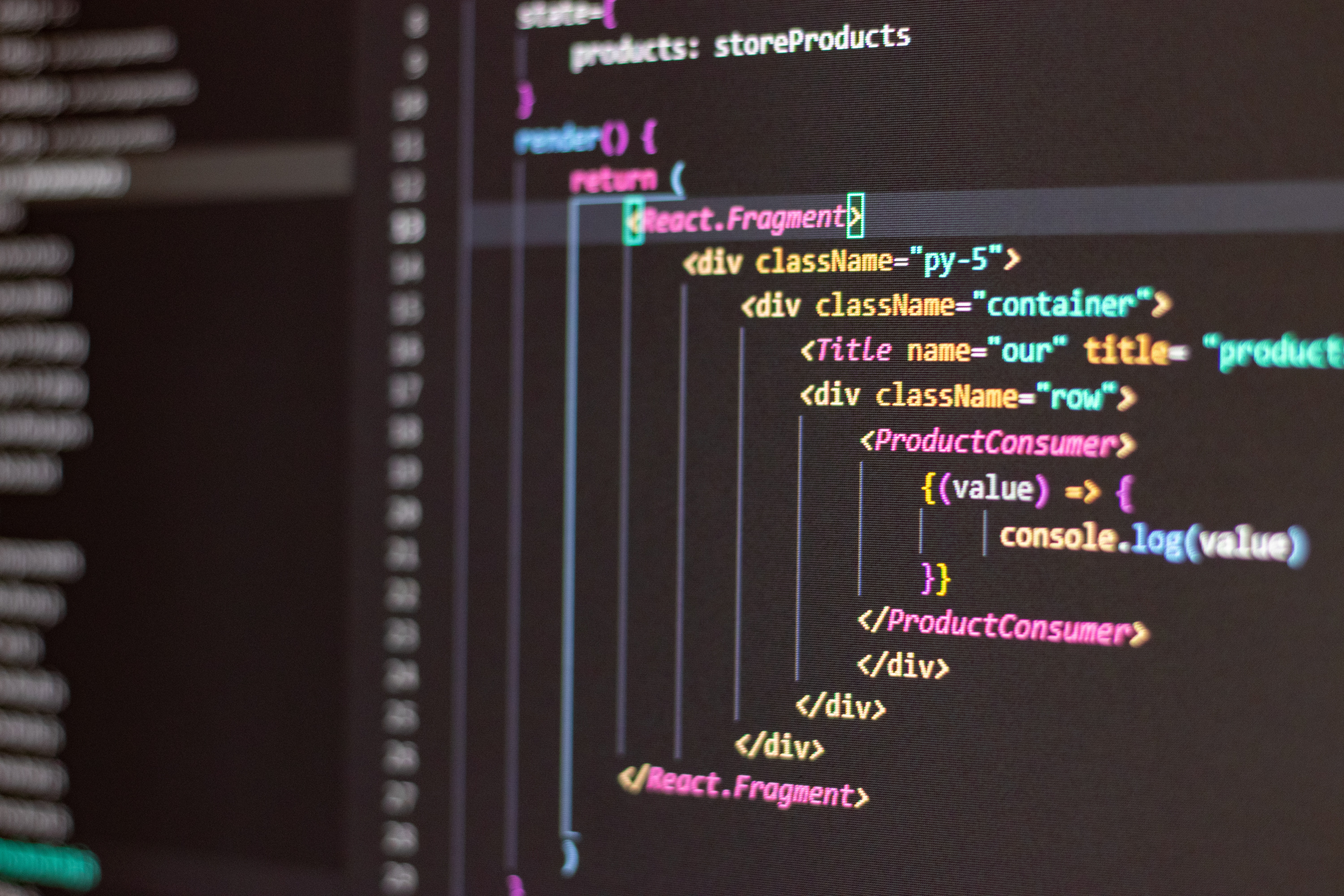
Asynchornous call is very famous for its callback hell
getData(a => { getMoreData(a, b => { getMoreData(b, c => { getMoreData(c, d => { getMoreData(d, e => { console.log(e); }); }); }); }); });
Promise Hell
getData() .then(a => getMoreData(a)) .then(b => getMoreData(b)) .then(c => getMoreData(c)) .then(d => getMoreData(d)) .then(e => console.log(e));
Its' not over yet
getData() .then(getMoreData) .then(getMoreData) .then(getMoreData) .then(getMoreData) .then(console.log);
Looks synchronous with async-await
(async () => { const a = await getData(); const b = await getMoreData(a); const c = await getMoreData(b); const d = await getMoreData(c); const e = await getMoreData(d); console.log(e); })